Prototype Design Pattern is duplicating objects using already created objects. Existing objects are used as a prototype. The intention of the Prototype Design Pattern is to create new objects using existing objects.
Normally create objects from the scratch, but considering the performance and the cost of generating objects sometimes it’s easy to copy the existing heavy objects.
There are objects that can be completed from a huge database process or after the bulk of network calls. But the next object works in the same way or object, not much change compared with the existing then creating a new object from the scratch is time-consuming and costly. Those situations are easily managed by coping with existing objects.
In the java clone()
the method used to clone the objects but developers feel free to use appropriate cloning mechanisms to use when copying objects. Prototype Design Pattern used to create a new object there for this pattern comes under creational design pattern.
Let’s take a real-world example of a Prototype Design Pattern.
Prototype Design Pattern Real World Example
We take an example of a car factory that we demonstrated on factory method design pattern. First, move into the class diagram of the example.
The normal flow inside the factory is following the same product structure to manufacture cars. There are minor changes in the outlook but implementation level, there are no major changes. Let’s check the class diagram.
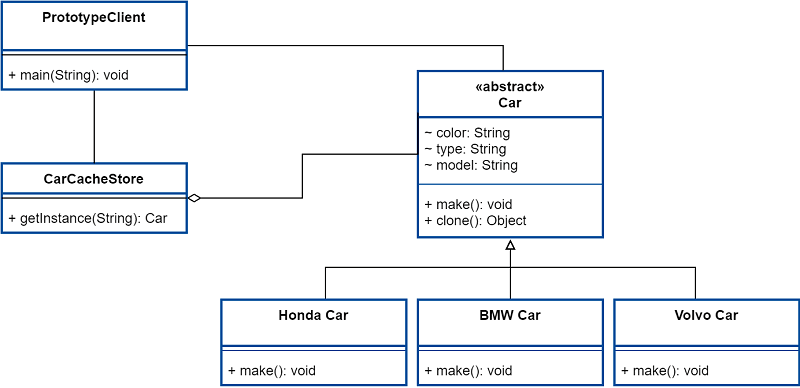
According to the class diagram, there are 3 participants in Prototype Design Pattern. Those are
- Prototype: Objects that going to copy
- Registry: Storage of the objects. Here we can use a caching mechanism to store objects
- Client: This is calling registry to create objects by clone.
Prototype Design Pattern in Java
import java.util.HashMap; import java.util.Map; // Define the basic car and all the basic structure of the car abstract class Car implements Cloneable{ private String color = null; private String type = null; private String model = null; public abstract void make(); public String getColor() { return color; } public void setColor(String color) { this.color = color; } public String getType() { return type; } public void setType(String type) { this.type = type; } public String getModel() { return model; } public void setModel(String model) { this.model = model; } // Clone the objects using Java in build functionality public Object clone(){ Object clone = null; try{ clone = super.clone(); } catch (CloneNotSupportedException e) { e.printStackTrace(); } return clone; } public String toString() { return "Car cloned and car creates including " +this.color+", "+this.model+", "+this.type+" features"; } } // Inherit basic Car and derive Clone from it class BmwCar extends Car{ @Override public void make() { System.out.println("made Honda car"); } } class HondaCar extends Car{ @Override public void make() { System.out.println("made Honda car"); } } class VolvoCar extends Car{ @Override public void make() { System.out.println("made Volvo car"); } } // Car Cache for retrive once copy needed. class CarCacheStore{ private static Map<String, Car> carMap = new HashMap<String, Car>(); static { Car bmwCar=new BmwCar(); bmwCar.setColor("Red"); bmwCar.setType("Auto"); bmwCar.setModel("BMW"); carMap.put("BMW", bmwCar); Car hondaCar=new HondaCar(); hondaCar.setColor("White"); hondaCar.setType("Auto"); hondaCar.setModel("HONDA"); carMap.put("HONDA", hondaCar); Car volvoCar=new VolvoCar(); volvoCar.setColor("Blue"); volvoCar.setType("Auto"); volvoCar.setModel("VOLVO"); carMap.put("VOLVO",volvoCar); } // Clone car Objects when required. All the concrete car objects derides clone() from Car // BmwCar, HondaCar, VolvoCar creates own copy of car by calling clone method public static Car getInstance(String carName) { return (Car) carMap.get(carName).clone(); } } public class PrototypeClient { // Once make a copy of car then minor modification easy to apply public static void main(String[] args) { // Get BMW car copy Car car1 = CarCacheStore.getInstance("BMW"); System.out.println(car1); // Get HONDA car copy Car car2 = CarCacheStore.getInstance("HONDA"); System.out.println(car2); // Get VOLVO car copy Car car3 = CarCacheStore.getInstance("VOLVO"); System.out.println(car3); } }
Output
Car cloned and car creates including Red, BMW, Auto features Car cloned and car creates including White, HONDA, Auto features Car cloned and car creates including Blue, VOLVO, Auto features
Advantages of Prototype Design Pattern
- If an Object with hierarchical then needing to build the whole structure again and again for the same structure is costly. But a clone is easy to use in that case.
- If one uses caching mechanism to store objects that is faster and good to use for distributable applications.
- Easy to extend programming: Objects are already created just need to extend the application using it. No need to worry about how to create it.
Summary
I think everyone as a programmer uses Clone objects in applications. That’s everywhere, there are mainly two types of cloning. Programmers need to decide the clone type according to the situation. Those are
- Shallow Clone
- Deep Clone
Prototype Design Pattern is heavily used in complex applications. It’s because patterns are making programming easier. We need to use them in the correct way, and we can’t use a single pattern at a time. Most of the time it’s a combination of patterns in the real world.
Design Patterns Book
Design Patterns are one of the most famous solutions in programing and millions of people follow them to fix their tasks, design projects, and so on. Most of the patterns create based on basic OOP concepts (If your beginner then read our basic OOP, Encapsulation, Inheritance, Polymorphism, and Abstraction articles with great examples )
There are a lot of articles and design pattern books available with different examples to read. Other than our article if you are interested in this topic you could go through the below books as well.
Books | Description |
---|---|
Head First Design Patterns: A Brain-Friendly Guide | My favorite and it’s more readable. There are good examples and they look good to beginners as well. |
Design Patterns: Elements of Reusable Object-Oriented Software | There are 23 design patterns. showing how to select an appropriate pattern for your case. |
Head First Design Patterns: Building Extensible and Maintainable Object-Oriented Software | There are a lot of simple examples showing how to use design patterns in the correct way. Those are based on SOLIC principles. |
There are a lot of design pattern ebooks on Amazon. Those design patterns are written with great examples in a different programming language. I included a few of them according to their best ranking.