Filter Design Pattern enables filtering the dataset according to specific criteria. Free to define different types of criteria and it enables users to add new criteria once it is needed. This pattern comes under a structural design pattern. If you like to see the Design pattern follow these publishers.
The pattern intention is to create a subset of the main dataset using filters. To apply this pattern there should be a dataset and filters with a filter mechanism. Let’s take an example in Real World.
Filter Design Pattern Real World Example
In-vehicle registration companies register vehicles and they need to filter vehicles according to different criteria. It can be vehicle type, Fuel type or manufactured year, and so on. Let’s implement the vehicle registration filters as loosely coupled.
First, move into a class diagram of vehicle registration.
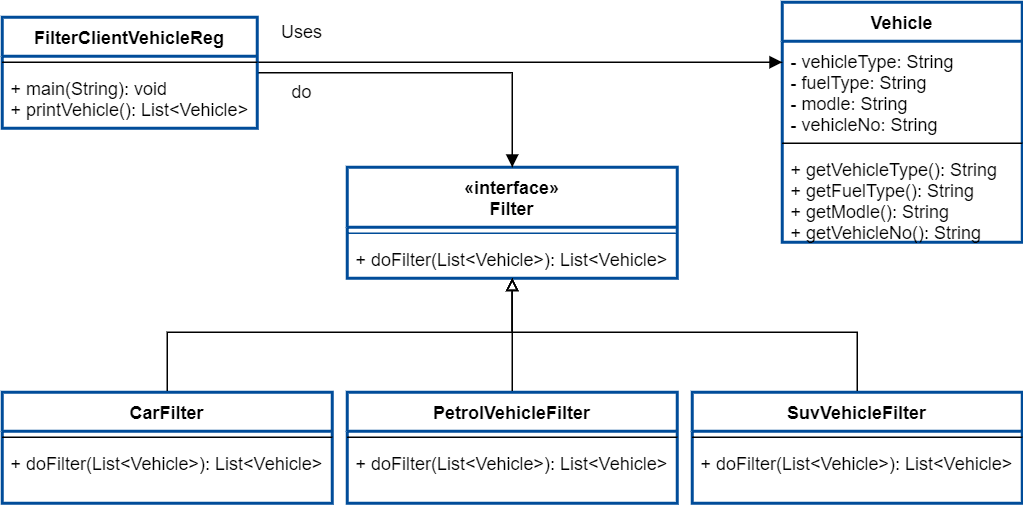
Filter Design Pattern in Java
According to the class diagram, there are three highlighted participants in the pattern
- Abstract Filter: Definition of filters (Interface)
- Concrete Filters: Implementation of different types of criteria
- Filter Object: Main object type for creating the master dataset
// Main object to create dataset class Vehicle{ private String vehicleType; private String fuelType; private String modle; private String vehicleNo; public Vehicle(String vehicleType, String fuelType, String modle,String vehicleNo) { this.vehicleType = vehicleType; this.fuelType = fuelType; this.modle = modle; this.vehicleNo=vehicleNo; } public String getVehicleType() { return vehicleType; } public String getFuelType() { return fuelType; } public String getModle() { return modle; } public String getVehicleNo() { return vehicleNo; } } // Definition of filters interface Filter{ List<Vehicle> doFilter(List<Vehicle> vehicles); } //Creates filter to filter the main dataset class CarFilter implements Filter{ public List<Vehicle> doFilter(List<Vehicle> vehicles){ List<Vehicle> car = new ArrayList<Vehicle>(); for (Vehicle vehicle : vehicles) { if (vehicle.getVehicleType().equals("Car")) { car.add(vehicle); } } return car; } } class PetrolVehicleFilter implements Filter{ public List<Vehicle> doFilter(List<Vehicle> vehicles){ List<Vehicle> petrolVehicle = new ArrayList<Vehicle>(); for (Vehicle vehicle : vehicles) { if (vehicle.getFuelType().equals("Petrol")) { petrolVehicle.add(vehicle); } } return petrolVehicle; } } class SuvVehicleFilter implements Filter{ public List<Vehicle> doFilter(List<Vehicle> vehicles){ List<Vehicle> suvVehicle = new ArrayList<Vehicle>(); for (Vehicle vehicle : vehicles) { if (vehicle.getModle().equals("SUV")) { suvVehicle.add(vehicle); } } return suvVehicle; } } public class FilterClientVehicleReg { public static void main(String[] args) { List<Vehicle> vehicles = new ArrayList<Vehicle>(); vehicles.add(new Vehicle("Car", "Petrol", "SUV","CP CAV 1234")); vehicles.add(new Vehicle("Bus", "Diesel", "Single deck","CP ND 5345")); vehicles.add(new Vehicle("Car", "Petrol", "SUV","WP CAB 9776")); vehicles.add(new Vehicle("Car", "Petrol", "HatchBack","NC GB 4367")); vehicles.add(new Vehicle("Bike", "Petrol", "Scooter","WP CAA 3467")); vehicles.add(new Vehicle("Car", "Diesel", "Saloon","NC HAK 3456")); vehicles.add(new Vehicle("Bus", "Diesel", "Minibus","WP NA 8977")); Filter carFilter= new CarFilter(); Filter petrolFilter= new PetrolVehicleFilter(); Filter suvFilter =new SuvVehicleFilter(); System.out.println("Filtered car list from the main set"); printVehicle(carFilter.doFilter(vehicles)); System.out.println("Filtered Petrol Vehicles from the main set"); printVehicle(petrolFilter.doFilter(vehicles)); System.out.println("Filtered SUV Type Vehicles from the main set"); printVehicle(suvFilter.doFilter(vehicles)); } public static void printVehicle(List<Vehicle> vehicles){ for (Vehicle vehicle : vehicles) { System.out.println("Vehicle "+vehicle.getVehicleNo()+ " informations are: {"+vehicle.getVehicleType() +" - "+vehicle.getModle()+" - "+vehicle.getFuelType()+"}"); } } }
Clients are free to use any type of filter according to their dataset. Also free to extend the code by adding any type of criteria to the system.
Output
Filtered car list from the main set Vehicle CP CAV 1234 informations are: {Car - SUV - Petrol} Vehicle WP CAB 9776 informations are: {Car - SUV - Petrol} Vehicle NC GB 4367 informations are: {Car - HatchBack - Petrol} Vehicle NC HAK 3456 informations are: {Car - Saloon - Diesel} Filtered Petrol Vehicles from the main set Vehicle CP CAV 1234 informations are: {Car - SUV - Petrol} Vehicle WP CAB 9776 informations are: {Car - SUV - Petrol} Vehicle NC GB 4367 informations are: {Car - HatchBack - Petrol} Vehicle WP CAA 3467 informations are: {Bike - Scooter - Petrol} Filtered SUV Type Vehicles from the main set Vehicle CP CAV 1234 informations are: {Car - SUV - Petrol} Vehicle WP CAB 9776 informations are: {Car - SUV - Petrol}
Advantages of Filter Design Pattern
- Filter dynamically list of data set
- Add new filters without affecting the rest of the code or filters
Filter Pattern In Java Core
Lambda Expressions in Java 8
Filter design pattern filtering dataset according to specific criteria there for a pattern also calls as criteria pattern. If you use Lambda Expressions in Java 8 you can use a filter pattern without knowing it’s the filter pattern. This is a simple example of a Lambda expression. But it’s not easy to understand by looking at it. we can write complex code in a less number of lines by using the lambda expression
import java.lang.FunctionalInterface; // we are creating simple functional interface @FunctionalInterface interface MySimpleInterface{ // abstract method double getMathPiValue(); } public class Main { public static void main( String[] args ) { // creating a reference to MySimpleInterface MySimpleInterface ref; // this usage of lambda expression ref = () -> 3.1415; System.out.println("Math Value of Pi = " + ref.getMathPiValue()); } }
Stream API in Java 8
Java Stream API is also a well-known library introduced by Java 8. This also heavily uses a filter pattern for filter datasets according to your criteria. Here I added simple code for filter employees according to specific criteria by using Stream API as a usage of filter pattern in Jaca Core libraries
public static void main(String... args) { Employee tom= new Employee ("Tom", Gender.MALE, MaritalStatus.MARRIED); Employee jerry= new Employee ("Jerry", Gender.FEMALE, MaritalStatus.MARRIED); Employee matt= new Employee ("Matt, Gender.MALE, MaritalStatus.SINGLE); Set<Employee> malemarried= Stream.of(tom, jerry, matt) .filter(person -> person.gender == Gender.MALE) .filter(person -> person.maritalStatus == MaritalStatus.MARRIED) .collect(Collectors.toSet()); } enum Gender { MALE, FEMALE } enum MaritalStatus { MARRIED, SINGLE } static class Employee{ final String name; final Gender gender; final MaritalStatus maritalStatus; Employee(String name, Gender gender, MaritalStatus maritalStatus){ this.name = name; this.gender = gender; this.maritalStatus = maritalStatus; }
Design Patterns Book
Design Patterns are one of the most famous solutions in programing and millions of people follow them to fix their tasks, design projects, and so on. Most of the patterns create based on basic OOP concepts (If your beginner then read our basic OOP, Encapsulation, Inheritance, Polymorphism, and Abstraction articles with great examples )
There are a lot of articles and design pattern books available with different examples to read. Other than our article if you are interested in this topic you could go through the below books as well.
Books | Description | |
---|---|---|
Head First Design Patterns: A Brain-Friendly Guide | My favorite and it’s more readable. There are good examples and they look good to beginners as well. | |
Design Patterns: Elements of Reusable Object-Oriented Software | There are 23 design patterns. showing how to select an appropriate pattern for your case. | |
Head First Design Patterns: Building Extensible and Maintainable Object-Oriented Software | There are a lot of simple examples showing how to use design patterns in the correct way. Those are based on SOLIC principles. |
There are a lot of design pattern ebooks on Amazon. Those design patterns are written with great examples in a different programming language. I included a few of them according to their best ranking.
1 thought on “Filter Design Pattern: (It’s Now Simple)”
Nice post. I learn something totally new and challenging on sites I stumbleupon every day. Its always interesting to read articles from other authors and practice a little something from other web sites.