Singleton Design Pattern is one of the easiest patterns to understand. This pattern comes under creational design patterns. Singleton Design Pattern’s main intention is to create only a single object throughout the application at run time. Give a global access point to a single object and use its entire application.
Make sure the below rules apply when creating a singleton pattern.
- Constructors should be private
- Static single instance (Object) for the class.
- Static method to return the instance.
Using the above rule there is no way to create objects from outside the class. The only way to create an instance using the class method. Keep in mind, that object values can change anywhere in the application and those values are equal if the object is accessed from anywhere in the application. That’s because of returning the same object through the static method.
There are two ways to create a singleton pattern.
- Lazy initialization: Initialize objects when required.
- Early Instantiation: Initialize the object when loading the class by a class loader.
Let’s take the real-world example and code for each type in the next section
Singleton Design Pattern Real-World Example
Let’s take an example of a President in a country. There should be a single object (President) for a country at a time. Values can change with time bean. But there is one and only one object that can create using the president class.
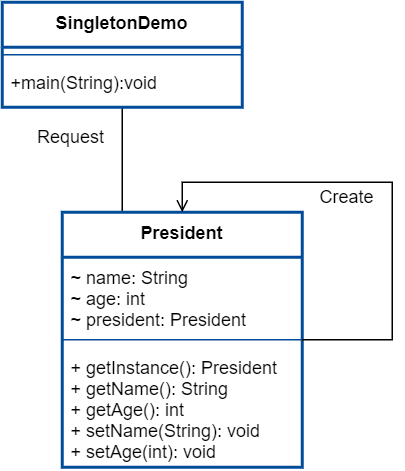
Singleton Design Pattern in Java
Early Instantiation
class President{ //private instanse early Instantiation private static President president=new President(); private String name; private int age; private President (){} // public method to return single instance public static President getInstance(){ return president; } public String toString(){ return "Name is "+name +" And Age is "+ age; } public void setName(String name){ this.name=name; } public void setAge(int age){ this.age=age; } public String getName(){ return name; } public int getAge(){ return age; } } public class SingletonDemo { public static void main(String[] args) { President president=President.getInstance(); president.setName("John Kalson"); president.setAge(56); System.out.println("Our President "+president); } }
In the example, the instance creates as Early Instantiation. Object access through the static method and there is no other way to use this class without the static instance.
Lazy initialization
class President{ //private instanse early Instantiation private static President president=new President(); private String name; private int age; private President (){} public String toString(){ return "Name is "+name +" And Age is "+ age; } public void setName(String name){ this.name=name; } public void setAge(int age){ this.age=age; } public String getName(){ return name; } public int getAge(){ return age; } // Lazy Instantiation of singleton pattern public synchronized static President getInstance(){ if (president == null) president=new President(); return president; } } public class SingletonDemo { public static void main(String[] args) { President president=President.getInstance(); president.setName("John Kalson"); president.setAge(56); System.out.println("Our President "+president); } }
output
Our President Name is John Kalson And Age is 56
There can be problems when in a singleton pattern if it is not implemented in the right way. Think about multitasking or multi-thread. We need to use the synchronization technique to make it thread-safe when lazy Instantiation. Also, we can use a key-locking mechanism when creating objects.
There are practical situations when programming with the singleton pattern. Our typical usage of a Database connection is unique and creates a single object for all the transactions. Another usage is configuration objects in the Spring Framework and Singleton is heavily used when auto-wiring in the spring framework.
Singleton Implementation Issues
- Make sure when lazy initialization of singleton object is thread-safe.
- We are expecting a single object for the entire JVM. But if there are different class loaders then there might be a situation loading static objects for each class loader.
Advantages of Singleton Design Pattern
- Memory usage is very less due to creating a single object for the entire application.
- Values can be set anywhere in the application
Design Patterns Book
Design Patterns are one of the most famous solutions in programming and millions of people follow them to fix their tasks, design projects, and so on. Most of the patterns create based on basic OOP concepts (If your beginner then read our basic OOP, Encapsulation, Inheritance, Polymorphism, and Abstraction articles with great examples )
There are a lot of articles and design pattern books available with different examples to read. Other than our article if you are interested in this topic you could go through the below books as well.
Books | Description |
---|---|
Head First Design Patterns: A Brain-Friendly Guide | My favorite and it’s more readable. There are good examples and they look good to beginners as well. |
Design Patterns: Elements of Reusable Object-Oriented Software | There are 23 design patterns. showing how to select an appropriate pattern for your case. |
Head First Design Patterns: Building Extensible and Maintainable Object-Oriented Software | There are a lot of simple examples showing how to use design patterns in the correct way. Those are based on SOLIC principles. |
There are a lot of design pattern ebooks on Amazon. Those design patterns are written with great examples in a different programming language. I included a few of them according to their best ranking.