Abstract Factory Design Pattern is one of the creational design patterns that make families of the dependent objects. This is also called the factory in factories and each factory connects with interfaces. Those interfaces are returning concrete class-type objects when clients request objects through the factory.
Abstract Factory Design Pattern built on top of the factory method pattern ( factory pattern ). Therefore Abstract Factory Design Pattern intention is similar to the factory method pattern and hides the logic from the client.
Its hides the mechanism of creating objects by giving a common interface to create or request objects. Users do not consider how factories produce objects, but just request what they need.
Abstract Factory Design Pattern Real World Example
Let’s take the vehicle building factory as an example. Consider Heavy vehicles develop in one factory and lightweight vehicles developed by another factory. Factory Provider is the front layer that creates contact with clients and provides services for them. But clients are not engaged directly with factories. Vehicle manufacturing inside the factories hides from clients.
Let’s go with a class diagram and try to understand how objects connect with factories.
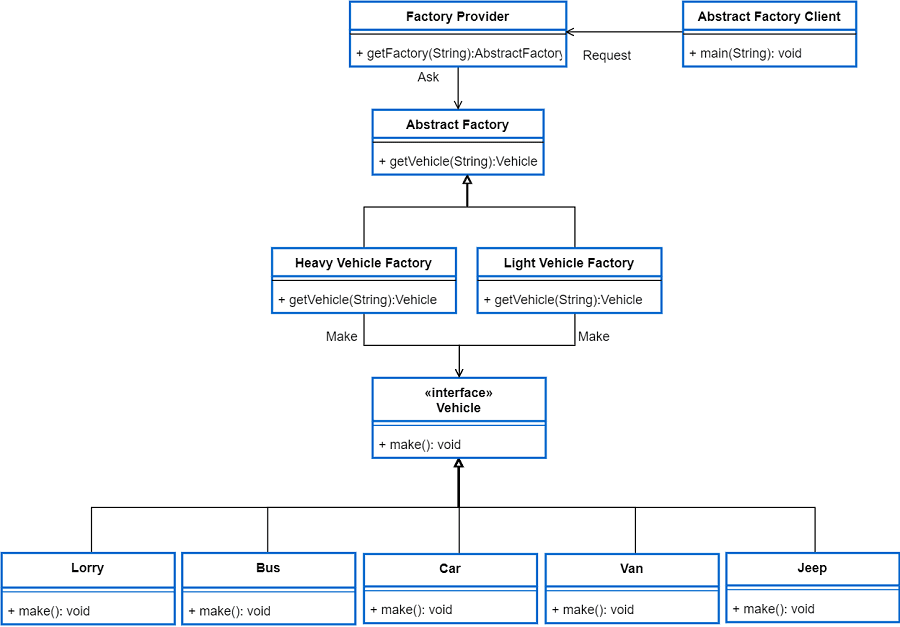
According to the diagram heavy vehicles build by a heavy vehicle factory and light vehicles build by another factory. But the client doing the deals with the Factory Provider. The factory provider is responsible for finding the correct factory and taking necessary actions for the client’s requests.
Then According to the diagram Client’s vehicle provide to the client through a factory. Keep in mind when going through the code and diagram all the concrete classes depend on interfaces or abstraction. That’s one of the good use of Design Principles.
Then move into to Java code implementation of the Abstract Factory Design Pattern.
Abstract Factory Design Pattern in Java
// Vehicles are independent. // New Vehicle start manufacturing in the system not break existing interface Vehicle{ void make(); } class Bus implements Vehicle{ @Override public void make() { System.out.println("Bus made"); } } class Lorry implements Vehicle{ @Override public void make() { System.out.println("Lorry made"); } } class Car implements Vehicle{ @Override public void make() { System.out.println("car made"); } } class Van implements Vehicle{ @Override public void make() { System.out.println("Van made"); } } class Jeep implements Vehicle{ @Override public void make() { System.out.println(" Jeep made"); } } abstract class AbstractFactory { abstract Vehicle getVehicle(String vehicleType) ; } // Factries are independent. New factory open to the system not break existing class HeavyVehicleFactory extends AbstractFactory{ public Vehicle getVehicle(String vehicleType){ if (vehicleType.equals("BUS")) { return new Bus(); } if (vehicleType.equals("LORRY")) { return new Lorry(); } return null; } } class LightVehicleFactory extends AbstractFactory{ public Vehicle getVehicle(String vehicleType){ if (vehicleType.equals("CAR")) { return new Car(); } if (vehicleType.equals("VAN")) { return new Van(); } if (vehicleType.equals("JEEP")) { return new Jeep(); } return null; } } // FactoryProvider looking for correct factory according to the clients requests class FactoryProvider { public AbstractFactory getFactory(String factory){ if(factory.equals("LIGHTVEHICLE")){ return new LightVehicleFactory(); } else if(factory.equals("HEAVYVEHICLE")){ return new HeavyVehicleFactory(); } return null; } } //Clients looking for vehicle but it calling throught the Provider. // Not direct contact with Factries.. public class AbstractFactoryClient { public static void main(String[] args) { FactoryProvider provider=new FactoryProvider(); AbstractFactory abstractFactory=provider.getFactory("LIGHTVEHICLE"); Vehicle vehicle=abstractFactory.getVehicle("VAN"); vehicle.make(); abstractFactory=provider.getFactory("HEAVYVEHICLE"); vehicle=abstractFactory.getVehicle("BUS"); vehicle.make(); } }
Output
Van made Bus made
Let’s consider if the company wants a new vehicle type to start production. Now all the existing vehicles depend on vehicle interface. If then-new production receives its easy to plug into the existing system without making any changes in existing code. Extending is very easy when depending on abstraction.
This extending concept applies not only to vehicles but also apply with factories. Opening a new factory is just extending an abstract factory.
Advantages of Abstract Factory Design Pattern
- Hide internal implementation of a factory and return client required through the interface.
- Loosely couple enables to extend the application without affecting other parties.
- Improve the security by loosely coupling.
Design Patterns Book
Design Patterns are one of the most famous solutions in programing and millions of people follow them to fix their tasks, design projects, and so on. Most of the patterns create based on basic OOP concepts (If your beginner then read our basic OOP, Encapsulation, Inheritance, Polymorphism, and Abstraction articles with great examples )
There are a lot of articles and design pattern books available with different examples to read. Other than our article if you are interested in this topic you could go through the below books as well.
Books | Description | |
---|---|---|
Head First Design Patterns: A Brain-Friendly Guide | My favorite and it’s more readable. There are good examples and they look good to beginners as well. | |
Design Patterns: Elements of Reusable Object-Oriented Software | There are 23 design patterns. showing how to select an appropriate pattern for your case. | |
Head First Design Patterns: Building Extensible and Maintainable Object-Oriented Software | There are a lot of simple examples showing how to use design patterns in the correct way. Those are based on SOLIC principles. |
There are a lot of design pattern ebooks on Amazon. Those design patterns are written with great examples in a different programming language. I included a few of them according to their best ranking.
2 thoughts on “What is Abstract Factory Design Pattern? (Easy)”
Hello would you mind stating which blog platform you’re working with?
I’m planning to start my own blog soon but I’m having a hard time making a decision between BlogEngine/Wordpress/B2evolution and Drupal.
The reason I ask is because your design seems different then most
blogs and I’m looking for something unique.
P.S Apologies for being off-topic but I had to ask!
Thank you for Reaching us https://ennicode.com/ , Yes this is done myself, and used Astra themes (https://wpastra.com/?bsf=10048) also used Elementer page builder (https://trk.elementor.com/liyl0yq1oh7n) for built website. Also if you like we can develop WordPress site for you (check our services: https://ennicode.com/services/). Thank you…