Overloading and Overriding in OOP (Object-Oriented Programming ) are frequently used object-oriented programming techniques. Those are used to write and design code clearly and smartly. Design patterns are heavily used overriding when designing the application.
What is Java Overloading?
In a single Java class possible to create methods with the same name but with different parameters. This is called method overloading. When method overloading, the compiler (Compile-time) is able to decide which method runs at runtime.
Method signature change according to the parameters in overloading. Therefore the compiler sees the difference between each method and decides which method to run. That’s called compile-time binding or static binding.
Java Overloading Rules
- Method name should be equal
- Parameter list should be different.
- Return type able to change in overloaded methods
- Access modifiers can change in overloaded methods.
- Exception may vary with overloaded methods
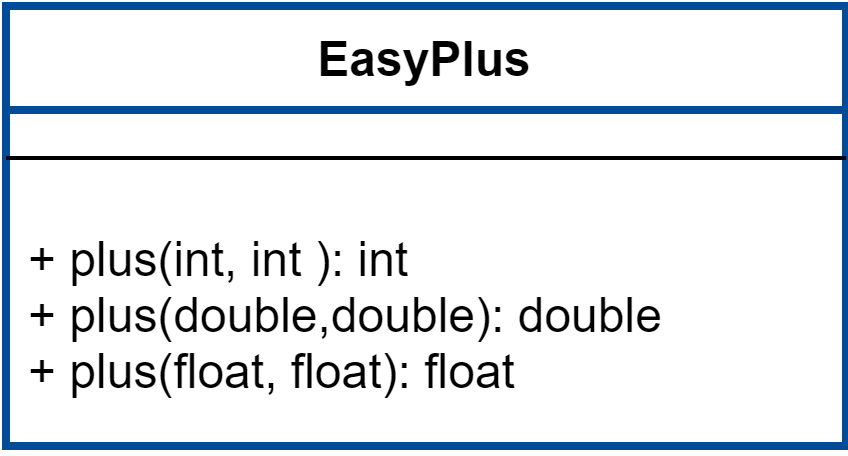
public class Overloading { public static void main(String[] args) { EasyPlus easyPlus =new EasyPlus(); System.out.println("2 + 3 = "+easyPlus.plus(2, 3)); System.out.println("2.5 + 3.5 = "+easyPlus.plus(2.5, 3.5)); System.out.println("2.5 + 3.5 = "+easyPlus.plus(2.5f, 3.5f)); } } // Created three functions with same name but with different parameters class EasyPlus{ public int plus(int a, int b){ return a+b; } public double plus(double a, double b){ return a+b; } public float plus(float a, float b){ return a+b; } }
Output
2 + 3 = 5 2.5 + 3.5 = 6.0 2.5 + 3.5 = 6.0
What is Java Overriding?
What Is Polymorphism In Object-Oriented Programming (OOP)? The page describes how polymorphism works. Run time or Dynamic polymorphism is through the inheritance. Inheritance (Look What Is Inheritance In Object-Oriented Programming (OOP)?) hierarchy subclass methods derive from a parent class and in subclass reimplement parent class method with the same signature is call method overriding.
Which overridden function to run at runtime is decided by JVM. It’s called runtime binding or dynamic binding. The overriding method should have the same signature (same name and same parameter). Reference of the object can be any parent type class of the inheritance hierarchy. But in the run time JVM runs only the object type method.
Java Overriding Rules
- Method signature must be equal (Name and Parameter List)
- Access modifiers can’t more restrictive. if the parent class method is “public” then Child class is not possible to “default, protected, or private”.
- Return type can be the same type or subtype as the parent class method.
- Overridden methods can throw any new unchecked exception.
- Overridden method can throw narrow or fewer checked exceptions but not throw any broader or wider exceptions than the parent method.
- Overriding only for inherited class methods
- Final methods are not allowed the override
- Static methods are not allowed to override but are able to redeclare.
- Constructors are not overriding.
Java Overriding Examples
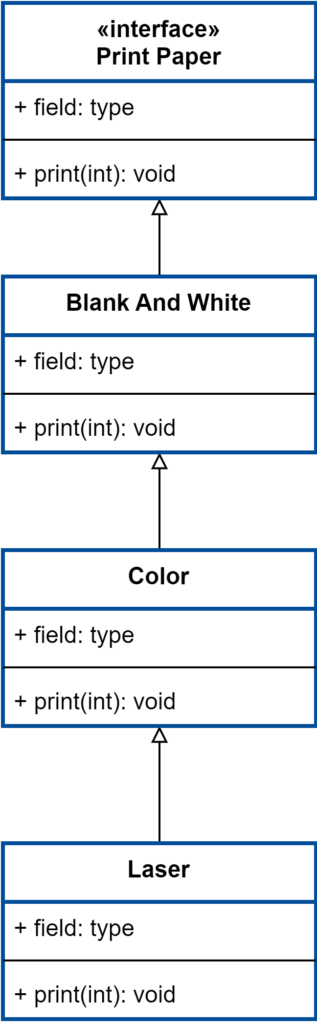
public class OverridingEx1 { public static void main(String[] args) { //Reference class is top of the hierarchy but objects type are child of PrintPaper PrintPaper printPaper; printPaper =new BlankAndWhite(); printPaper.print(1); printPaper =new Color(); printPaper.print(1); printPaper =new Laser(); printPaper.print(1); // Object in the runtime dicide which method to run } } //Define the print method in interface interface PrintPaper{ public void print(int paperCount); } // Method override for Black and white printing class BlankAndWhite implements PrintPaper{ public void print(int paperCount){ System.out.println("Print Black and White"); } } // Method override for color printing class Color extends BlankAndWhite{ public void print(int paperCount){ System.out.println("Print Color"); } } // Method override for laser color printing class Laser extends Color{ public void print(int paperCount){ System.out.println("Print Laser Color"); } }
Output
Print Black and White Print Color Print Laser Color
Java Overriding Dynamic Binding Extended
The Second example shows printMyPaper(PrintPaper printPaper)
receiving PrintPaper type object as a parameter but we can pass any type of object in the hierarchy. When runtime decides which method in class should be run.
public class OverridingEx2 { public static void main(String[] args) { OverridingEx2 overridingEx2 =new OverridingEx2(); overridingEx2.printMyPaper(new BlankAndWhite()); overridingEx2.printMyPaper(new Color()); overridingEx2.printMyPaper(new Laser()); } // printMyPaper method accept any type of object in Inheritance hierarchy public void printMyPaper(PrintPaper printPaper){ // JVM decide which method to run (Runtime Binding) printPaper.print(1); } }
Output
Print Black and White Print Color Print Laser Color
13 thoughts on “What Is Use Overloading and Overriding In OOP? Easy Examples”
I needed to thank you for this wonderful read!! I definitely enjoyed every bit of it. I have got you bookmarked to look at new things you post…
Thank you
Wonderful blog! I found it while surfing around on Yahoo News. Do you have any suggestions on how to get listed in Yahoo News? I’ve been trying for a while but I never seem to get there! Cheers
Thank you for Reaching us https://ennicode.com/ , I Just added records (Indexed) through the AllInOneSeo (WordPress Plugin). How to create indexing on this plugin. If you have time please share us with your friends.Thanks
Howdy! Someone in my Facebook group shared this site with us so I came to check it out. I’m definitely loving the information. I’m bookmarking and will be tweeting this to my followers! Great blog and excellent style and design.
Thank you for Reaching us https://ennicode.com/ , If you have time, share us with your friends Thanks
Its such as you learn my thoughts! You seem to grasp so much about this, such as you wrote the ebook in it or something. I believe that you just can do with a few percent to power the message home a bit, but instead of that, that is magnificent blog. A fantastic read. I will certainly be back.
Thank you for Reaching us https://ennicode.com/, If you have time, help us to share with your friends if you wish to receive new posts please subscribe. Thanks
Thanks for your post. I’d like to comment that the cost of car insurance varies greatly from one plan to another, simply because there are so many different issues which play a role in the overall cost. One example is, the brand name of the car or truck will have a significant bearing on the fee. A reliable aged family car will have a less expensive premium than just a flashy expensive car.
Thank you for Reaching us https://ennicode.com/, If you have time, help us to share with your friends if you wish to receive new posts please subscribe. Thanks
Hello my friend! I wish to say that this post is amazing, nice written and include almost all important infos. I?d like to see more posts like this.
Thank you for Reaching us https://ennicode.com/, If you have time, help us to share with your friends if you wish to receive new posts please subscribe. Thanks
Wow, fantastic weblog layout! How lengthy have you ever been running a blog for?
you make running a blog look easy. The overall look of
your site is fantastic, let alone the content material! You can see
similar: e-commerce and here sklep online