Factory Design Pattern or Factory Method Design Pattern’s main intention is to hide the implementation logic from the client. This is one of the famous patterns that comes under creational design patterns. The factory design pattern motivation is to loosely couple with client requirements (Front end) and implementation Logic(low-level).
When analyzing the pattern it looks pattern follows the SOLID principles correctly. For that Interfaces or Abstract, classes use to hide implantation from the backend. Design pattern helps to introduce new products to the factory without affecting the client. It maps with the Open Close principle in OOP programming.
Factory Design Pattern hides the object’s creation mechanism by giving a common interface to accept requests. Users do not consider how factories produce the product (here its objects) but just need what they requested.
Factory Design Pattern Real World Example
Today we are going to implement a car factory. For easiness think about one factory building BMW, Honda, and Volvo cars. Car factory’s main intention is to build cars according to customer trends. Once a client requests a BMW car and then the factory design and builds a car to supply the requirement. Clients do not bother about the manufacturing procedure of BMWs.
First, take the class diagram before moving into the source code.
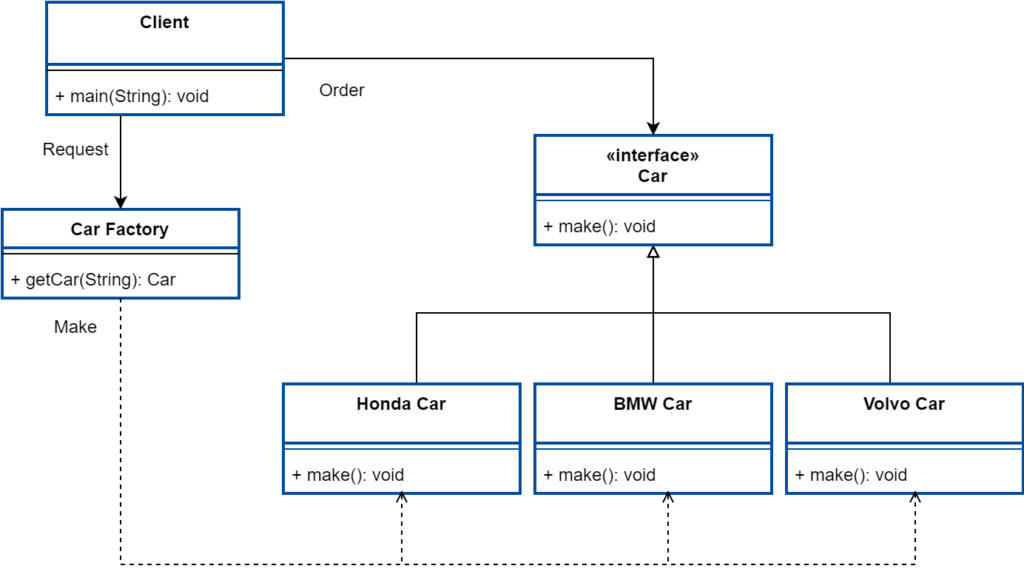
According to the diagram, the factory creates objects, and the clients are waiting for objects from the factory. Clients do not connect directly with real car objects but connect with an interface. Let’s move on to the implementation of the factory pattern.
Factory Design Pattern in Java
In the example, the main Interface is the Car class, and BMWCar, HondaCar, and VolovoCar classes create by implementing the Car interface through the inheritance. Factory returning Car but those instances created using subclasses of Car. That means Factory decides which class is used to make instances.
// Client one who requesting cars public class Client { public static void main(String[] args) { CarFactory factory=new CarFactory(); Car bmwCar=factory.getCar("BMW"); Car hondaCar=factory.getCar("HONDA"); Car volvoCar=factory.getCar("VOLVO"); bmwCar.make(); hondaCar.make(); volvoCar.make(); } } // Abstract car interface Car{ void make(); } class BmwCar implements Car{ @Override public void make() { System.out.println("made BMW car"); } } class HondaCar implements Car{ @Override public void make() { System.out.println("made Honda car"); } } class VolvoCar implements Car{ @Override public void make() { System.out.println("made Volvo car"); } } // CarFactory supplies according to the requests. New Cartype added to car CarFactory class CarFactory { public Car getCar(String carType){ if (carType.equals("BMW")) { return new BmwCar(); } if (carType.equals("HONDA")) { return new HondaCar(); } if (carType.equals("VOLVO")) { return new VolvoCar(); } return null; } }
Output
made BMW car made Honda car made Volvo car
Consider in later factory decide to build Mitsubishi cars or whatever, but there is still not any effect with clients. Just need to extend (Implement) the Car interface and add a new type to the factory. That means the factory can extend without modifying existing and without effect with clients and vice versa. It calls loosely coupling.
Advantages of Factory Design Pattern
- Hide internal implementation of a factory and return client requirements through the interface.
- Loosely couple enables to extend the application without affecting other parties
- Improve the security by Loosely coupling
Design Patterns Book
Design Patterns are one of the most famous solutions in programing and millions of people follow them to fix their tasks, design projects, and so on. Most of the patterns create based on basic OOP concepts (If your beginner then read our basic OOP, Encapsulation, Inheritance, Polymorphism, and Abstraction articles with great examples )
There are a lot of articles and design pattern books available with different examples to read. Other than our article if you are interested in this topic you could go through the below books as well.
Books | Description |
---|---|
Head First Design Patterns: A Brain-Friendly Guide | My favorite and it’s more readable. There are good examples and they look good to beginners as well. |
Design Patterns: Elements of Reusable Object-Oriented Software | There are 23 design patterns. showing how to select an appropriate pattern for your case. |
Head First Design Patterns: Building Extensible and Maintainable Object-Oriented Software | There are a lot of simple examples showing how to use design patterns in the correct way. Those are based on SOLIC principles. |
There are a lot of design pattern ebooks on Amazon. Those design patterns are written with great examples in a different programming language. I included a few of them according to their best ranking.