Strategy Design Pattern allows a user to change the context or behavior of an algorithm at runtime. There are situations from time to time that change the running behavior(algorithm ) according to the client’s context.
Also, there can be situations running behavior by adding a new algorithm to the system. In Both cases problem is to change the algorithm at runtime.
Strategy Design Pattern solves these types of problems. The pattern comes under a behavioral design pattern. Strategy Design Pattern is a good example of the Open Close Design Principle because the pattern allows (Open) users to add new algorithms without modifying the context.
Let’s move to the Participants of this pattern. There are 3 main participants in this pattern. those are
- Abstract Strategy: Definition of algorithm or definition of the operation
- Implementation of Strategy: Implementation of abstract Strategy. Implementation of Strategy objects used to change the algorithm of runtime.
- Context: Strategies are used by context and determine the implementation of strategies.
Next section let’s have a look at how patterns are used in the real world.
Strategy Design Pattern Real World Example
Consider there is a Bank that has different types of loan schemes by different conditions. For this example consider there are three loan schemes including different rates, loan insurance, guaranty conditions, etc. According to the scheme, there are different limits, and clients are able to select one of the loan schemes by their preference.
Clients have to select one out of three options as their loan and the bank will execute the selected scheme when processing. That means executing the loan scheme and conditions decided at runtime. The processing loan algorithm changes according to the selected loan type.
Also, if there is a requirement to add another fourth loan scheme to the system that’s easy to plug without affecting existing. Let’s move on to the class diagram and implementation example in Java.
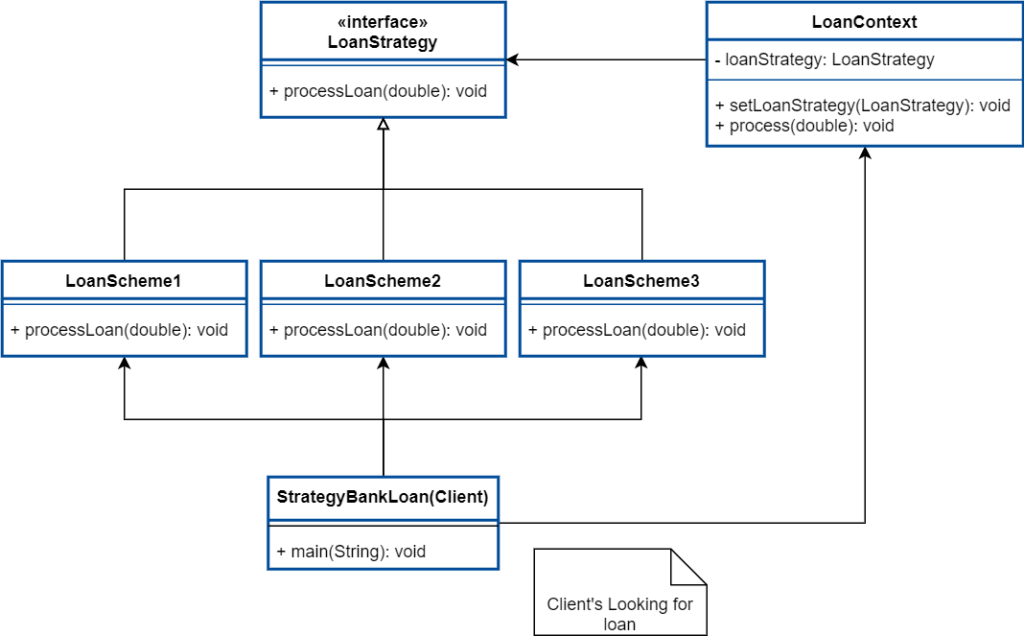
Strategy Design Pattern in Java
// Define the operations for the process loans interface LoanStrategy{ void processLoan(double amount); } // Implements the algorithm according the loan conditions // Conditions are change in each loan // and algorithm change according to that (Loan Stratagies) class LoanScheme1 implements LoanStrategy{ public void processLoan(double amount){ System.out.println("Loan Scheme 1 including 5% rate, No insurance, without guarantees for amount of "+amount); } } class LoanScheme2 implements LoanStrategy{ public void processLoan(double amount){ System.out.println("Loan Scheme 2 including 6% rate, with insurance, with 1 guarantee for amount of "+amount); } } class LoanScheme3 implements LoanStrategy{ public void processLoan(double amount){ System.out.println("Loan Scheme 3 including 7% rate, with insurance, with 2 guarantee for amount of "+amount); } } // Context use one of the strategy at runtime // Once select the strategy and process at runtime class LoanContext{ private LoanStrategy loanStrategy; public void setLoanStrategy(LoanStrategy loanStrategy) { this.loanStrategy = loanStrategy; } public void process(double amount) { loanStrategy.processLoan(amount); } } // Clients feel free to select one of the loan category // public class StrategyBankLoan { public static void main(String[] args) { LoanContext loanContext= new LoanContext(); loanContext.setLoanStrategy(new LoanScheme1()); loanContext.process(10000); loanContext.setLoanStrategy(new LoanScheme2()); loanContext.process(500000); loanContext.setLoanStrategy(new LoanScheme3()); loanContext.process(2000000); } }
Output
Loan Scheme 1 including 5% rate, No insurance, without guarantees for amount of 10000.0 Loan Scheme 2 including 6% rate, with insurance, with 1 guarantee for amount of 500000.0 Loan Scheme 3 including 7% rate, with insurance, with 2 guarantee for amount of 2000000.0
Advantages of Strategy Design Pattern
- New algorithms introduce and plug into a system without affecting the whole.
- Following the Open-close principle improves the maintainability.
- A client can choose an algorithm at runtime.
- Minimize the modification of code
Design Patterns Book
Design Patterns are one of the most famous solutions in programming and millions of people follow them to fix their tasks, design projects, and so on. Most of the patterns are created based on basic OOP concepts (If you beginner then read our basic OOP, Encapsulation, Inheritance, Polymorphism, and Abstraction articles with great examples )
There are a lot of articles and design pattern books available with different examples to read. Other than our article if you are interested in this topic you could go through the below books as well.
Books | Description |
---|---|
Head First Design Patterns: A Brain-Friendly Guide | My favorite and it’s more readable. There are good examples and they look good to beginners as well. |
Design Patterns: Elements of Reusable Object-Oriented Software | There are 23 design patterns. showing how to select an appropriate pattern for your case. |
Head First Design Patterns: Building Extensible and Maintainable Object-Oriented Software | There are a lot of simple examples showing how to use design patterns in the correct way. Those are based on SOLIC principles. |
There are a lot of design pattern ebooks on Amazon. Those design patterns are written with great examples in a different programming language. I included a few of them according to their best ranking.