Flyweight Design Pattern is to reduce the creates new objects by reusing or sharing the already created objects. Some application needs to create a large number of similar objects, but objects with few attributes also behave in the same way. Flyweight Design Pattern reduces memory by reusing already created objects.
Flyweight Design Pattern comes under structural patterns. The main intention of the Flyweight Design Pattern is to reuse the objects from existing ones if it is needed. Keep in mind Flyweight applies to the immutable object. There are two kinds of attributes referred to here.
- Intrinsic: If object-related attributes can share with other objects and those are similar to each other, then attributes are in an Intrinsic state.
- Extrinsic: Some attributes are not similar to each other or attributes are defined from a client then those attributes in an Extrinsic state.
Let’s clear Flyweight Design Pattern with intrinsic and extrinsic attributes using a class diagram and example.
Flyweight Design Pattern Real World Example
Consider five trains arrange and set ready to travel from one location to another location. But only one train travels in one day. Considering train travel route, passenger count, and no of stops are equal with every five trains when start traveling then those types of attributes is an intrinsic state.
But consider train driver and train number are not sharable with each other. Also, those are not similar to each other. Those attributes call as extrinsic attributes.
When considering picking randomly one train each day out of five trains to travel the entire month. Once trains are in a ready state and according to the Flyweight Design Pattern just pick a train out of five and move to run (Running state). Because all the trains are in a ready-to-go state. Let’s see the diagram
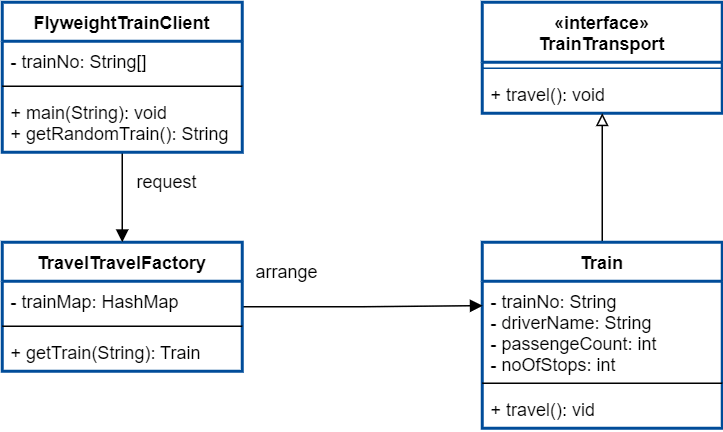
Flyweight Design Pattern in Java
// Train default function as travel interface TrainTransport{ void travel(); } // Main class for create object to share class Train implements TrainTransport{ private String trainNo; //extrinsic attribute private String driverName; //extrinsic attribute private int passengeCount; //intrinsic attribute private int noOfStops; //intrinsic attribute public Train(String trainNo) { this.trainNo=trainNo; } @Override public void travel() { System.out.println("Train Driver "+driverName+ " is start the Train No "+trainNo+" to travel"); } public void setTrainNo(String trainNo) { this.trainNo = trainNo; } public void setDriverName(String driverName) { this.driverName = driverName; } public void setPassengeCount(int passengeCount) { this.passengeCount = passengeCount; } public void setNoOfStops(int noOfStops) { this.noOfStops = noOfStops; } @Override public String toString() { return "Train No "+trainNo+" is arrange and ready to travel"; } } class TravelTravelFactory{ private static final HashMap<String,Train> trainMap = new HashMap<String,Train>(); // Facotry create and put into map/cache and return new/existing train public static Train getTrain(String trainNo) { Train train = trainMap.get(trainNo); if(train == null) { train = new Train(trainNo); train.setNoOfStops(20); train.setPassengeCount(1000); trainMap.put(trainNo, train); System.out.println(""+train); } return train; } } public class FlyweightTrainClient { private static final String trainNo[] = { "Ek102", "HM399", "DF304", "SL234", "BM987" }; public static void main(String[] args) { YearMonth yearMonthObject = YearMonth.of(2021, 11); int daysInMonth = yearMonthObject.lengthOfMonth(); // Travel entire month for (int i = 0; i < daysInMonth; i++) { String trainNo=getRandomTrain(); // Pick randomly selected train to travel Train train = TravelTravelFactory.getTrain(trainNo); train.setDriverName("TrainDriverFor"+trainNo); train.travel(); } } // Get random train private static String getRandomTrain() { return trainNo[(int)(Math.random()*trainNo.length)]; } }
According to the code, trains are set ready and keep it on the list. In this case able to use a caching mechanism to keep objects. Once the object required to pick those are available in the cache.
Output
Train No DF304 is arrange and ready to travel Train Driver TrainDriverForDF304 is start the Train No DF304 to travel Train No Ek102 is arrange and ready to travel Train Driver TrainDriverForEk102 is start the Train No Ek102 to travel Train No HM399 is arrange and ready to travel Train Driver TrainDriverForHM399 is start the Train No HM399 to travel Train Driver TrainDriverForDF304 is start the Train No DF304 to travel Train No SL234 is arrange and ready to travel Train Driver TrainDriverForSL234 is start the Train No SL234 to travel Train Driver TrainDriverForDF304 is start the Train No DF304 to travel Train Driver TrainDriverForEk102 is start the Train No Ek102 to travel Train Driver TrainDriverForEk102 is start the Train No Ek102 to travel Train No BM987 is arrange and ready to travel Train Driver TrainDriverForBM987 is start the Train No BM987 to travel Train Driver TrainDriverForHM399 is start the Train No HM399 to travel Train Driver TrainDriverForBM987 is start the Train No BM987 to travel Train Driver TrainDriverForSL234 is start the Train No SL234 to travel Train Driver TrainDriverForEk102 is start the Train No Ek102 to travel Train Driver TrainDriverForBM987 is start the Train No BM987 to travel Train Driver TrainDriverForEk102 is start the Train No Ek102 to travel Train Driver TrainDriverForHM399 is start the Train No HM399 to travel Train Driver TrainDriverForBM987 is start the Train No BM987 to travel
Advantages of Flyweight Design Pattern
- Reduce the number of object creation
- Memory usage reduces by sharing objects
- Reduce object creation time
Design Patterns Book
Design Patterns are one of the most famous solutions in programing and millions of people follow them to fix their tasks, design projects, and so on. Most of the patterns create based on basic OOP concepts (If your beginner then read our basic OOP, Encapsulation, Inheritance, Polymorphism, and Abstraction articles with great examples )
There are a lot of articles and design pattern books available with different examples to read. Other than our article if you are interested in this topic you could go through the below books as well.
Books | Description | |
---|---|---|
Head First Design Patterns: A Brain-Friendly Guide | My favorite and it’s more readable. There are good examples and they look good to beginners as well. | |
Design Patterns: Elements of Reusable Object-Oriented Software | There are 23 design patterns. showing how to select an appropriate pattern for your case. | |
Head First Design Patterns: Building Extensible and Maintainable Object-Oriented Software | There are a lot of simple examples showing how to use design patterns in the correct way. Those are based on SOLIC principles. |
There are a lot of design pattern ebooks on Amazon. Those design patterns are written with great examples in a different programming language. I included a few of them according to their best ranking.