State Design Pattern is introduced as an easy way of handling behaviors and actions of a specific object according to its current state. Once change the state of the object it behaves in a different way. State Design Pattern helps to maintain the flow of the life cycle by using state machines.
This is a behavioral design pattern and there are three main components in the pattern.
- State: The state defines the operation needed to be performed in each state.
- Concrete State: Concrete implementation of State and perform the operation.
- Context: Keep the current state by referencing the Concrete State Object.
According to the above components, an object starts creating in an initial state, and then the state changes and saves the current state inside the Context. We can use the current state of an object when performing different actions through the application.
State Design Pattern Real World Example
Consider the manufacturing industry. Some states are handling when production. Think about car manufacturing. It starts with the Design state next moves into the Implementation state after that cars move to the Test state next into the Shipping state and finally cars in the Delivered state. This is a simple example flow of manufacturing.
There are different functioning that happens in each state. But calling each function decides according to the current state of the product. Therefore state handling rather than using many IF ELSE condition make code clean and understandable. Let’s move on to the class diagram and implementation of this example.
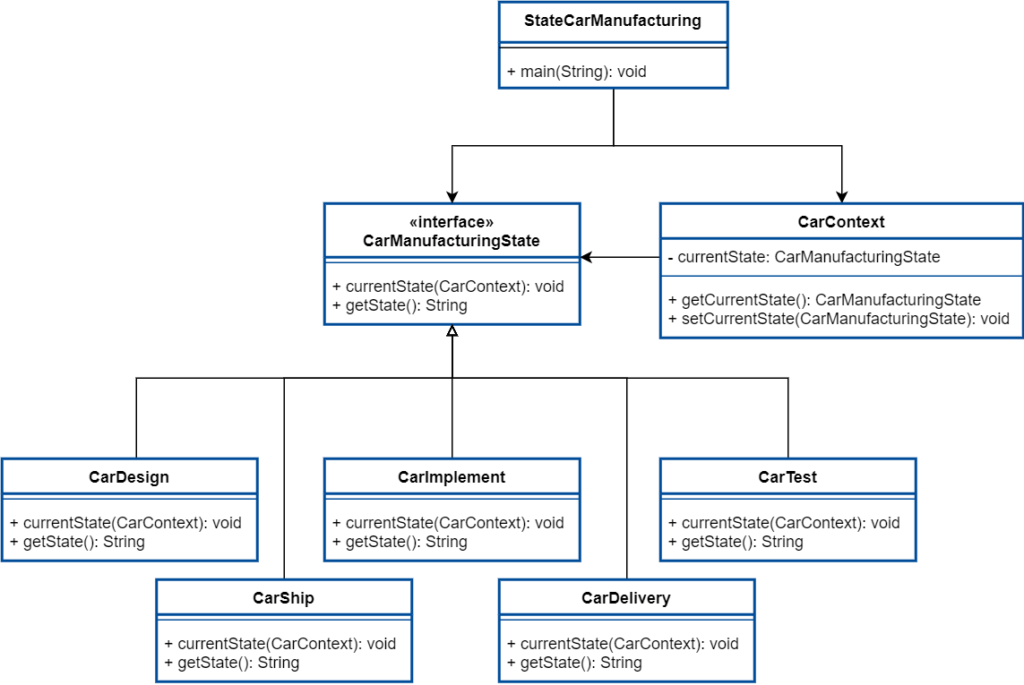
State Design Pattern in Java
Think about according to the below example manufacturing state will change once done specified things with the car. But if we need to add new functionality in Test Phrase then just need to check the state of the car.
//(Abstract State) // Interface for define state of action interface CarManufacturingState{ void currentState(CarContext carContext); String getState(); } // (Concreate State) // There are 5 Concrete State in this example when manufacturing car class CarDesign implements CarManufacturingState{ public void currentState(CarContext carContext){ carContext.setCurrentState(this); } public String getState(){ return "Design"; } } class CarImplement implements CarManufacturingState{ public void currentState(CarContext carContext){ carContext.setCurrentState(this); } public String getState(){ return "Implementation"; } } class CarTest implements CarManufacturingState{ public void currentState(CarContext carContext){ carContext.setCurrentState(this); } public String getState(){ return "Test"; } } class CarShip implements CarManufacturingState{ public void currentState(CarContext carContext){ carContext.setCurrentState(this); } public String getState(){ return "Shipping"; } } class CarDelivery implements CarManufacturingState{ public void currentState(CarContext carContext){ carContext.setCurrentState(this); } public String getState(){ return "Delivery"; } } // (Context) // Context class for keep the current state of manufacturing class CarContext{ private CarManufacturingState currentState; public CarManufacturingState getCurrentState() { return currentState; } public void setCurrentState(CarManufacturingState currentState) { this.currentState = currentState; } } public class StateCarManufacturing { public static void main(String[] args) { CarContext carContext = new CarContext(); CarDesign carDesign = new CarDesign(); carDesign.currentState(carContext); System.out.println("Car Current state is: " +carContext.getCurrentState().getState()); CarImplement carImplement = new CarImplement(); carImplement.currentState(carContext); System.out.println("Car Current state is: " +carContext.getCurrentState().getState()); CarTest carTest = new CarTest(); carTest.currentState(carContext); System.out.println("Car Current state is: " +carContext.getCurrentState().getState()); CarShip carShip = new CarShip(); carShip.currentState(carContext); System.out.println("Car Current state is: " +carContext.getCurrentState().getState()); CarDelivery carDelivery = new CarDelivery(); carDelivery.currentState(carContext); System.out.println("Car Current state is: " +carContext.getCurrentState().getState()); } }
Output
Car Current state is: Design Car Current state is: Implementation Car Current state is: Test Car Current state is: Shipping Car Current state is: Delivery
Advantages of State Design Pattern
- Easy to add new state middle of the process.
- States hide and return the current state of runtime.
- Reduce the IF condition and complexity of the code
Design Patterns Book
Design Patterns are one of the most famous solutions in programming and millions of people follow them to fix their tasks, design projects, and so on. Most of the patterns create based on basic OOP concepts (If your beginner then read our basic OOP, Encapsulation, Inheritance, Polymorphism, and Abstraction articles with great examples )
There are a lot of articles and design pattern books available with different examples to read. Other than our article if you are interested in this topic you could go through the below books as well.
Books | Description |
---|---|
Head First Design Patterns: A Brain-Friendly Guide | My favorite and it’s more readable. There are good examples and they look good to beginners as well. |
Design Patterns: Elements of Reusable Object-Oriented Software | There are 23 design patterns. showing how to select an appropriate pattern for your case. |
Head First Design Patterns: Building Extensible and Maintainable Object-Oriented Software | There are a lot of simple examples showing how to use design patterns in the correct way. Those are based on SOLIC principles. |
There are a lot of design pattern ebooks on Amazon. Those design patterns are written with great examples in a different programming language. I included a few of them according to their best ranking.