Observer Design Pattern is one of the heavily used patterns in social media. It’s because someone creates or modified something in their channel, website, or anywhere that message(Notifications) will notify their all followers. The theory of this mechanism describes under Observer Design Pattern.
If there is a One-to-Many relationship between objects and one object modifies then the rest of the object gets updated by automatic notification. But all dependents get notifications automatically if objects follow the subject.
Observer Design Pattern comes under behavioral pattern and This is working a similar way of Publisher Subscriber.
There are two main components in this pattern Subject (Publisher) and Observers (Subscriber). But there are three participants in this pattern. Those are
- Subject: Observers register with Subject and Publishing messages with registered observers.
- Observer: Interface or abstract definition and define the operations to be notified.
- Concrete observer: Implementation of Observer gets notifications from Subject.
According to the above participants, once the Subject publishes something then, a notification receives to observers saying the status of the Subject changed. Observers are able to register and unregister with the Subject and Observers need to register themselves with the subject to get notification from the Subject.
Let’s take real-world examples to understand how messages pass with observers.
Observer Design Pattern Real World Example
Consider you create an account and registering for notification with a bank. After registration, they will send notifications to registered clients related to changes in the bank. It may be a new loan scheme, new credit card offers and etc.
In this example, bank clients are the observers and the bank is the subject. According to your satisfaction, you can register or unregister for notification with the bank. Bank sends notifications as a message, email, or any other way of communication. Let’s implement our example with a class diagram.
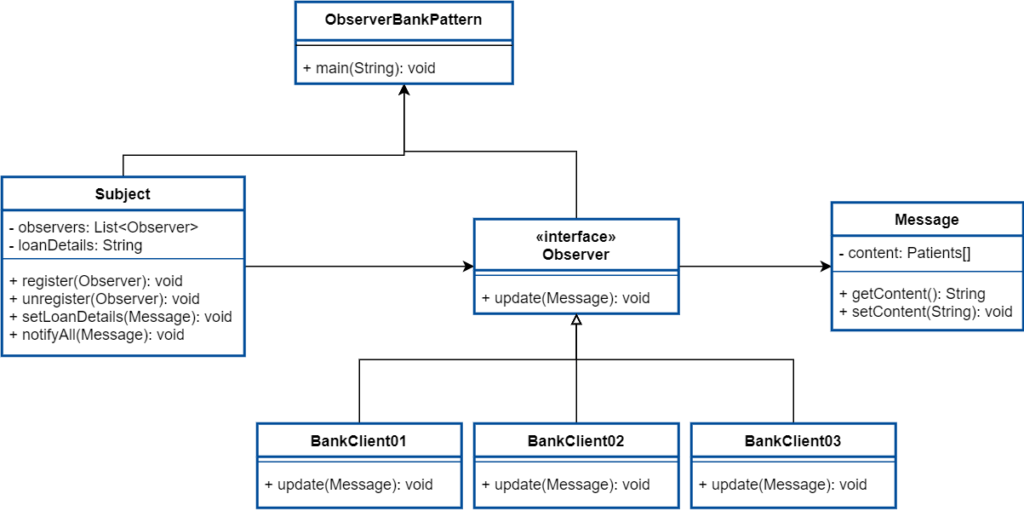
Observer Design Pattern in Java
//Subject Or Publisher change their status and notify with all class Subject{ private List<Observer> observers = new ArrayList<>(); private String loanDetails; public void register(Observer observer){ observers.add(observer); } public void unregister(Observer observer){ observers.remove(observer); } public void setLoanDetails(Message message) { this.loanDetails = loanDetails; notifyAll(message); } public void notifyAll(Message message){ for(Observer o: observers) { o.update(message); } } } // Abstract Observer use for loosy couple interface Observer{ public void update(Message message); } // Observer 1 or Subscriber 1 get notification from subject class BankClient01 implements Observer{ public void update(Message message){ System.out.println("Observer Bank Client 01: "+message.getContent()); } } // Observer 2 or Subscriber 2 get notification from subject class BankClient02 implements Observer{ public void update(Message message){ System.out.println("Observer Bank Client 02: "+message.getContent()); } } // Observer 3 or Subscriber 3 get notification from subject class BankClient03 implements Observer{ public void update(Message message){ System.out.println("Observer Bank Client 03: "+message.getContent()); } } // Use to pass the Notifications class Message{ private String content; public String getContent() { return content; } public void setContent(String content) { this.content = content; } } public class ObserverBankPattern { public static void main(String[] args) { BankClient01 bankClient01= new BankClient01(); BankClient02 bankClient02= new BankClient02(); BankClient03 bankClient03= new BankClient03(); Subject subject= new Subject(); subject.register(bankClient01); subject.register(bankClient02); subject.register(bankClient03); Message message1= new Message(); message1.setContent("Reduce loan rate by 1% from today onwards"); subject.setLoanDetails(message1); Message message2= new Message(); message2.setContent("Introducing New housing load scheme for professionals"); subject.setLoanDetails(message2); } }
Output
Observer Bank Client 01: Reduce loan rate by 1% from today onwards Observer Bank Client 02: Reduce loan rate by 1% from today onwards Observer Bank Client 03: Reduce loan rate by 1% from today onwards Observer Bank Client 01: Introducing New housing load scheme for professionals Observer Bank Client 02: Introducing New housing load scheme for professionals Observer Bank Client 03: Introducing New housing load scheme for professionals
Advantages of Observer Design Pattern
- Subject and Observers are loosely coupled.
- Attach or detach observers without knowing the Subject.
- Observers are independent of their work not any forces from a subject.
Design Patterns Book
Design Patterns are one of the most famous solutions in programing and millions of people follow them to fix their tasks, design projects, and so on. Most of the patterns create based on basic OOP concepts (If your beginner then read our basic OOP, Encapsulation, Inheritance, Polymorphism, and Abstraction articles with great examples )
There are a lot of articles and design pattern books available with different examples to read. Other than our article if you are interested in this topic you could go through the below books as well.
Books | Description | |
---|---|---|
Head First Design Patterns: A Brain-Friendly Guide | My favorite and it’s more readable. There are good examples and they look good to beginners as well. | |
Design Patterns: Elements of Reusable Object-Oriented Software | There are 23 design patterns. showing how to select an appropriate pattern for your case. | |
Head First Design Patterns: Building Extensible and Maintainable Object-Oriented Software | There are a lot of simple examples showing how to use design patterns in the correct way. Those are based on SOLIC principles. |
There are a lot of design pattern ebooks on Amazon. Those design patterns are written with great examples in a different programming language. I included a few of them according to their best ranking.