Adapter Design Pattern is working as a connector of non-compatible interfaces. That means converting one interface into another interface expectation. In the real-world Adapter is used to connect two pieces of equipment that do not have a direct connection and also it is used to make connections between them.
The Adapter Design Pattern comes under structural design patterns. Let’s take a few examples to clear the adapter pattern.
Adapter Design Pattern Real World Example
Example 1
USB card readers use to read memory cards for computers. There are a lot of power adapters used to plug the equipment into the power.
Example 2
Think about a smart type of television that does not have an AV port but supports HDMI Video input. You have an old laptop with AV out and you want to play MP4 on the big screen on the television. The solution is an adapter. That means AV to HDMI converting adapter is used to solve the problem.
Let’s try to implement the problem using java. Therefore first go through the class diagram.
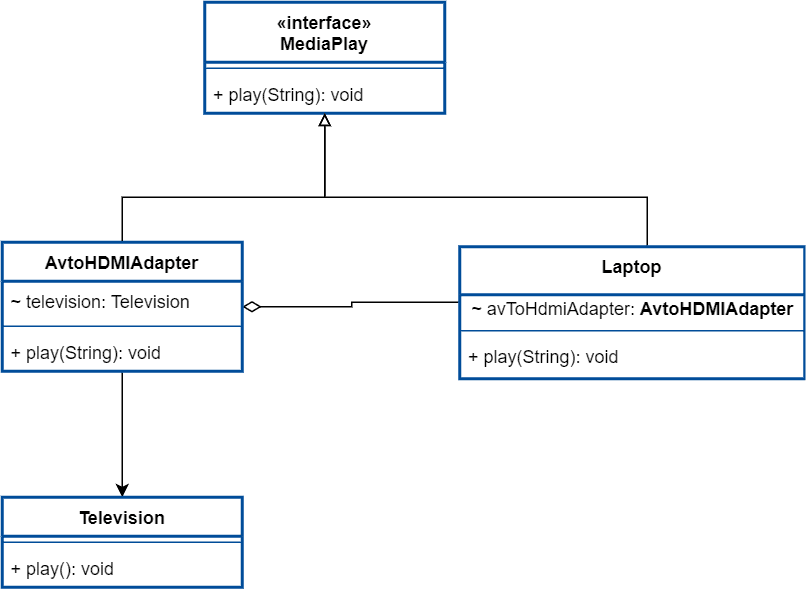
Adapter Design Pattern in Java
According to the diagram, there are four main participants in the Adapter Design Pattern. Those are
- Adapter: The class used to connect two non-matching interfaces (AvtoHdmiAvadaptor)
- Adaptee: This class is used by the Adapter class. which is going to execute functionality according to the adapter. (Television with Av)
- Client: Directly connect with the Adapter to complete work through the Adapter. (Laptop)
- Interface: Abstract functionality that going to implement. (MediaPlay)
According to the implementation, the Adapter support playing video on television. If the Adapter supports more than one type that will add in the future without any modification in other classes.
// Media player is the Interface define main functionality interface MediaPlay{ public void play(String playMode); } // Television play only HDMI input format class Television { public void play() { System.out.println("Play video in Television with HDMI input."); } } // Laptop play itself or play in TV through the Adapter class Laptop implements MediaPlay{ AvToHDMIAdapter avToHDMIAdapter; @Override public void play(String playMode) { if (playMode.equals("Laptop")) { System.out.println("Play video in the Laptop."); } // Need to use the HDMI adapter to play and convert Av to HDMI else if (playMode.equals("TV")) { avToHDMIAdapter= new AvToHDMIAdapter(); avToHDMIAdapter.play("TV"); } } } // Adapter play and convert video to HDMI class AvToHDMIAdapter implements MediaPlay{ private Television television; public AvToHDMIAdapter() { television= new Television(); } public void play(String playMode) { // Adapter play video in TV if(playMode.equals("TV")){ television.play(); } //IF Adapter Support with another Equipment its comes here (If Projector) } } public class AdapterClient { public static void main(String[] args) { MediaPlay mediaPlay=new Laptop(); // Play only in Laptop mediaPlay.play("Laptop"); // Play with TV mediaPlay.play("TV"); } }
Output
Play video in the Laptop. Play video in Television with HDMI input.
Advantages of Adapter Design Pattern
- Code reusability that does not interconnect.
- Make compatibility with an individual object that does not have a direct connection between them.
Summary
The pattern creates a bridge between two classes or two objects. The adapter design pattern uses by java core libraries. They are
- java.util.Arrays#asList()
- java.io.OutputStreamWriter(OutputStream)
java.io.InputStreamReader(InputStream)
Design Patterns Book
Design Patterns are one of the most famous solutions in programing and millions of people follow them to fix their tasks, design projects, and so on. Most of the patterns create based on basic OOP concepts (If your beginner then read our basic OOP, Encapsulation, Inheritance, Polymorphism, and Abstraction articles with great examples )
There are a lot of articles and design pattern books available with different examples to read. Other than our article if you are interested in this topic you could go through the below books as well.
Books | Description |
---|---|
Head First Design Patterns: A Brain-Friendly Guide | My favorite and it’s more readable. There are good examples and they look good to beginners as well. |
Design Patterns: Elements of Reusable Object-Oriented Software | There are 23 design patterns. showing how to select an appropriate pattern for your case. |
Head First Design Patterns: Building Extensible and Maintainable Object-Oriented Software | There are a lot of simple examples showing how to use design patterns in the correct way. Those are based on SOLIC principles. |
There are a lot of design pattern ebooks on Amazon. Those design patterns are written with great examples in a different programming language. I included a few of them according to their best ranking.