Interpreter Design Pattern provides an interpreter to deal with grammar in programming languages. Interpreter Design Pattern predefines the rules for the grammar of a language. This pattern comes under a behavioral design pattern and the pattern is used in SQL parsing and Symbol processing.
Let’s see how Interpreter Design Pattern behaves. Consider some contexts receive into the pattern and pattern interpret the context using expressions. Expressions are in a specific hierarchy and those hierarchies of expressions are arranged like a tree structure. A tree creates leaves and roots in the same fashion, Expression may be leaf Expressions or root Expressions.
Leaf Expressions call as Terminal expressions and non-leafs are Non-Terminal expressions. According to the interpreter design pattern, there are 4 main participants. These are terminal Expressions, Non-terminal Expressions, Context, and Clients. If you remember the Composite design pattern this is also something similar too, but not exactly.
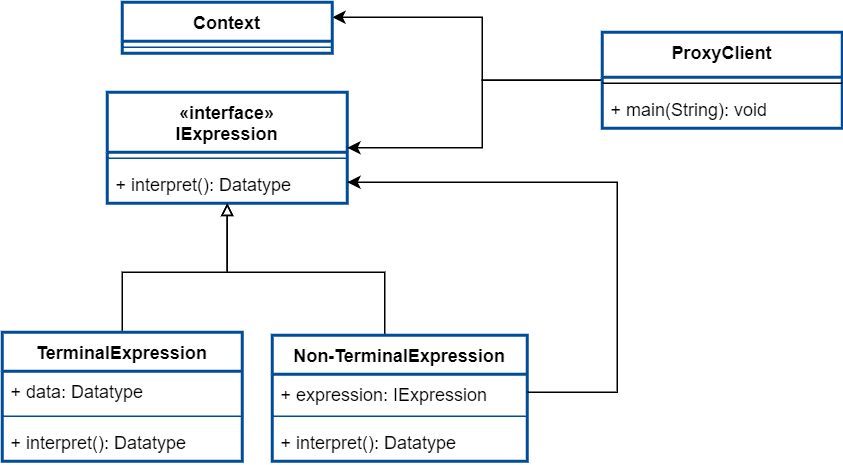
There are 5 class elements in Interpreter Design Pattern. Let’s investigate those
- Abstract Expressions: Define the interpreter method that will override concrete expressions.
- Terminal Expression: Main interpreter of context. Complete the interpreting by Terminal Expression and return the final output.
- Non-terminal Expressions: Recursively interpret and create combinational expressions.
- Context: This is the thing that needs to interpret.
- Clients: Invoker of the interpreter.
Let’s move into the Real World Example to make it clearer.
Interpreter Design Pattern Real World Example
Consider the musician playing the guitar by looking at notations. Those notations are just like a collection of signs. But musicians are able to make those notations into to musical beat or song. That means the musician interpreting those notations into that beat. According to Interpreter Design Pattern, the musician is the interpreter here.
In this case, there are a lot of notations and sign-in music. Therefore let’s take a simple example in electronics to implement in our case. Logical gates are basic building blocks for digital circuits. Perform logical functions and return certain output. In this example going to use NOT, AND, and OR gates as Non-terminal Expressions.
Create context as an input to the pattern then expect specific output from it. Let’s move into the class diagram to design the example of logical gates. Client sending context as logical input and gates doing interpretation. Final output return by the terminal expression.
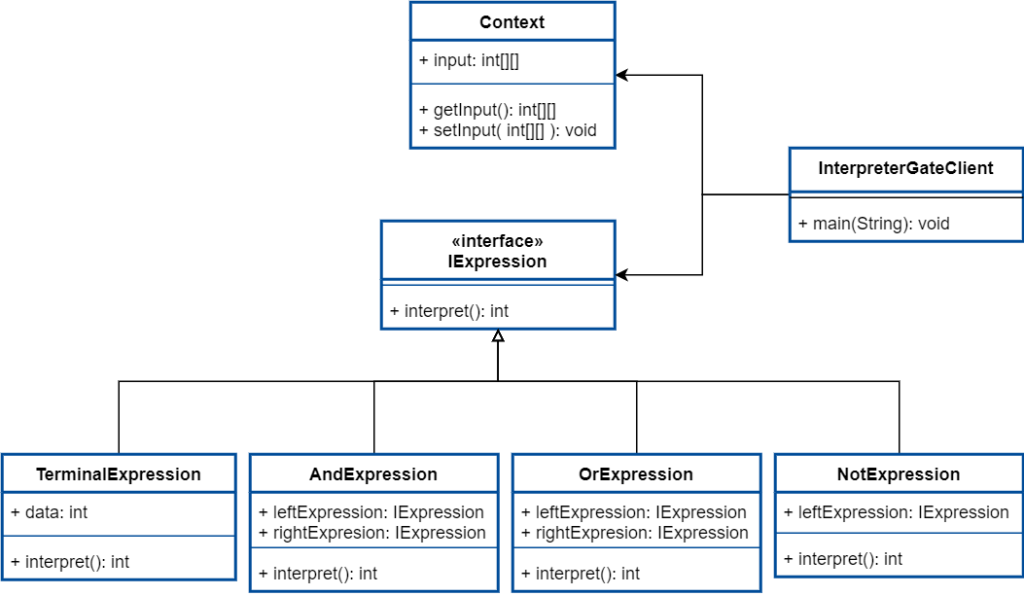
Interpreter Design Pattern in Java
// Expression Interface interface IExpression{ int interpret(); } // Terminal Expression class TerminalExpression implements IExpression{ int data; public TerminalExpression(String data) { this.data = Integer.parseInt(data); } public TerminalExpression(int data){ this.data = data; } public int interpret(){ if(data==1){ return 1; } else{ return 0; } } } // Concrete Non Terminal Expression (Logical AND Gate) class AndExpression implements IExpression { IExpression leftExpression; IExpression rightExpresion; public AndExpression(IExpression leftExpression, IExpression rightExpresion) { this.leftExpression = leftExpression; this.rightExpresion = rightExpresion; } @Override public int interpret() { return leftExpression.interpret() * rightExpresion.interpret(); } } // Concrete Non Terminal Expression (Logical OR Gate) class OrExpression implements IExpression { IExpression leftExpression; IExpression rightExpresion; public OrExpression(IExpression leftExpression, IExpression rightExpresion) { this.leftExpression = leftExpression; this.rightExpresion = rightExpresion; } @Override public int interpret() { return leftExpression.interpret()+ rightExpresion.interpret(); } } // Concrete Non Terminal Expression (Logical NOT Gate) class NotExpression implements IExpression { IExpression iExpression; public NotExpression(IExpression iExpression) { this.iExpression = iExpression; } @Override public int interpret() { if (iExpression.interpret()==1) { return 0; }else{ return 1; } } } class Context{ int[][] input = { {0,0}, {0,1}, {1,0}, {1,1} }; // Input Context public int[][] getInput() { return input; } public void setInput(int[][] input) { this.input = input; } } // Client invoke the expression public class InterpreterGateClient { // _ //OutPut= AB+B // Main Context to create input //| A | B | Out | //| 0 | 0 | 1 | //| 0 | 1 | 0 | //| 1 | 0 | 1 | //| 1 | 1 | 1 | public static void main(String[] args) { int[][] input = new Context().getInput(); for (int A = 0; A < input.length; ++A) { // AND expression - AB IExpression andGate = getAndExpression(input[A][0], input[A][1]); int andResult = andGate.interpret(); // _ // NOT expression - B IExpression notGate = getNotExpression(input[A][1]); int notResult = notGate.interpret();// // _ // OR expression - AB+B IExpression orGate = getOrExpression(andResult,notResult); int finalResult = orGate.interpret(); System.out.println("Final Result is "+finalResult); } } //Create AND exression Class public static IExpression getAndExpression(int left,int right){ IExpression leftExpression = new TerminalExpression(left); IExpression rightExpresion = new TerminalExpression(right); return new AndExpression(leftExpression, rightExpresion); } //Create OR exression Class public static IExpression getOrExpression(int left,int right){ IExpression leftExpression = new TerminalExpression(left); IExpression rightExpresion = new TerminalExpression(right); return new OrExpression(leftExpression, rightExpresion); } //Create NOT exression Class public static IExpression getNotExpression(int left){ IExpression leftExpression = new TerminalExpression(left); return new NotExpression(leftExpression); } }
Output
Final Result is 1 Final Result is 0 Final Result is 1 Final Result is 1
Conclusion
Interpreter Design Pattern provides an interpreter to deal with grammar. This pattern suit grammar that is not complicated. If grammar is complex, then this is going to be a lot of classes and maybe a problem with maintainability. Therefore Interpreter Design Pattern is suited to simple grammar and it’s straightforward.
Design Patterns Book
Design Patterns are one of the most famous solutions in programing and millions of people follow them to fix their tasks, design projects, and so on. Most of the patterns create based on basic OOP concepts (If your beginner then read our basic OOP, Encapsulation, Inheritance, Polymorphism, and Abstraction articles with great examples )
There are a lot of articles and design pattern books available with different examples to read. Other than our article if you are interested in this topic you could go through the below books as well.
Books | Description | |
---|---|---|
Head First Design Patterns: A Brain-Friendly Guide | My favorite and it’s more readable. There are good examples and they look good to beginners as well. | |
Design Patterns: Elements of Reusable Object-Oriented Software | There are 23 design patterns. showing how to select an appropriate pattern for your case. | |
Head First Design Patterns: Building Extensible and Maintainable Object-Oriented Software | There are a lot of simple examples showing how to use design patterns in the correct way. Those are based on SOLIC principles. |
There are a lot of design pattern ebooks on Amazon. Those design patterns are written with great examples in a different programming language. I included a few of them according to their best ranking.
7 thoughts on “Interpreter Design Pattern: A simple Converter”
Nice post. I learn something totally new and challenging on sites I stumbleupon every day. Its always interesting to read articles from other authors and practice a little something from other web sites.
Good post. I learn something new and challenging on sites I stumbleupon every day. It will always be exciting to read through content from other writers and use a little something from other sites.
Thank you for Reaching us https://ennicode.com/, If you have time, share us with your friends if you wish to receive new posts please subscribe Thanks
Everything is very open with a clear clarification of the issues. It was really informative. Your site is very useful. Thanks for sharing!
Thank you for Reaching us https://ennicode.com/, If you have time, share us with your friends if you wish to receive new posts please subscribe Thanks
This is the perfect blog for everyone who would like to find out about this topic. You know a whole lot its almost hard to argue with you (not that I actually would want toÖHaHa). You definitely put a fresh spin on a subject which has been discussed for ages. Wonderful stuff, just excellent!
Thank you for Reaching us https://ennicode.com/, If you have time, share us with your friends if you wish to receive new posts please subscribe Thanks