Chain of Responsibility Design Pattern is processing client or sender requests through a chain of handlers. On the receiving side, there is a chain of handlers that handle different types of requests. The client will pass a request without knowing the handler’s information, but the receiver decides how to process a request by themselves.
Clients’ requests are processed by a single handler or else multiple handlers or a subset of handlers. Request pass between handlers until completely handled by matching handler/s. Each handler there is connected with the next handler according to a planned sequence.
This type of pattern comes under behavioral patterns (Design Patterns). One of the objectives of the pattern is to decouple the sender and receiver. Once who needs to handle a request with multiple objects/components then this pattern prefers to use.
There are three components in the Chain of Responsibility Design Pattern
- Handler: Most of the time this is going to be an Interface/Abstract class and primary receive point to the request. This is the reference type of the first handler but doesn’t know about the rest of the handlers. Then it dispatches with concrete handlers.
- Concrete Handler: Chain of handlers that will handle the requests.
- Client/ Sender: Origin of the request
Let’s move on to a Real-World Example with the Chain of Responsibility Design Pattern
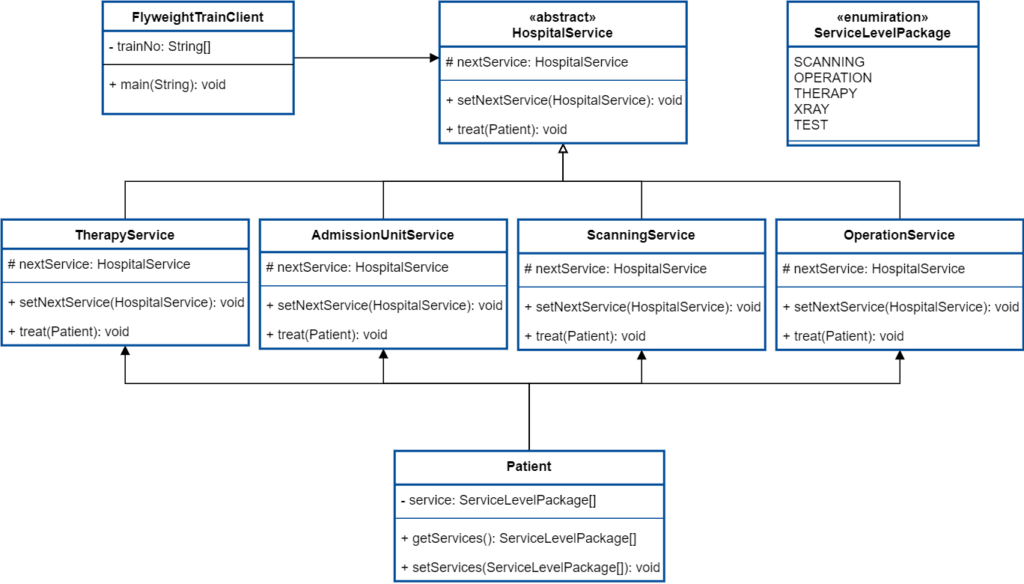
Chain of Responsibility Design Pattern Real World Example
There is a chain of responsibilities in day-to-day work life. Offices, Schools, Service centers, Hospitals, and a lot of places maintain responsibilities as a chain. Let’s take the hospital as our example for further discussion.
There are medical packages in hospitals for personal medical checkups. Once we are admitted to the hospital for a checkup through the admission center. The patient or guardian not going to do anything until discharged patient from the hospital. But there are a lot of processes happening in hospitals to look after and treat them. Patients may move to Scans, Operations, Therapies, and a lot more.
Hospitals do the treating to a patient as part of the whole responsibility chain or single person responsibility. That is decided by the hospital, but not by the patient or guardian. Eventually, discharge the patient once the hospital treatments. Responsibilities are different in each section and those who are doing what they have to do as a duty.
Let’s move on to the Class diagram to demonstrate the above scenario.
Chain of Responsibility Design Pattern in Java
According to the diagram, let’s build the pattern. Patterns move the patient from an initial location to an end until the treatments. Each Service connects to the next service. Once one service is complete then the patient moves into the next service according to the chain. Service check that the patient needs the current service, then treatment or service given to the patient.
// // Services supporting by the hospital enum ServiceLevelPackage { SCANNING, OPERATION, THERAPY, XRAY, TEST } // Admit Patient to hospital class Patient { private ServiceLevelPackage[] service; public ServiceLevelPackage[] getServices() { return service; } public void setServices(ServiceLevelPackage[] service) { System.out.println("Services added to patient"); this.service = service; } } // Abstract service as initial point abstract class HospitalService{ protected HospitalService nextService; public void setNextService(HospitalService nextService) { this.nextService = nextService; } abstract void treat(Patient patient); } // Start the service from AdmissionUnitService class AdmissionUnitService extends HospitalService{ public void treat(Patient patient){ if(nextService !=null){ nextService.treat(patient); } } } // ScanningService is one of the service by hospital class ScanningService extends HospitalService{ public void treat(Patient patient){ for (ServiceLevelPackage type : patient.getServices()) { if (type.equals(ServiceLevelPackage.SCANNING)) { System.out.println("Complete Scanning Service"); } } if(nextService !=null){ nextService.treat(patient); } } } // OperationService is one of the service by hospital class OperationService extends HospitalService{ public void treat(Patient patient){ for (ServiceLevelPackage type : patient.getServices()) { if (type.equals(ServiceLevelPackage.OPERATION)) { System.out.println("Complete Operation Service"); } } if(nextService !=null){ nextService.treat(patient); } } } // TherapyService is one of the service by hospital class TherapyService extends HospitalService{ public void treat(Patient patient){ for (ServiceLevelPackage type : patient.getServices()) { if (type.equals(ServiceLevelPackage.THERAPY)) { System.out.println("Complete Therapy Service"); } } if(nextService !=null){ nextService.treat(patient); } } } // Client looking for service from the hospital public class ChainOfResponsabilityPatient { public static void main(String[] args) { HospitalService admissionUnitService = new AdmissionUnitService(); HospitalService scanningService = new ScanningService(); HospitalService therapyService = new TherapyService(); HospitalService operationService = new OperationService(); admissionUnitService.setNextService(scanningService); scanningService.setNextService(therapyService); therapyService.setNextService(operationService); Patient patient = new Patient(); patient.setServices(new ServiceLevelPackage[]{ ServiceLevelPackage.SCANNING, ServiceLevelPackage.THERAPY, ServiceLevelPackage.OPERATION}); admissionUnitService.treat(patient); } }
Output
Services added to patient Complete Scanning Service Complete Therapy Service Complete Operation Service
Advantages of Chain of Responsibility Design Pattern
- Loosely couple Receive and Sender.
- Adding a new service to the chain does not affect with rest of the system.
- Dynamically handle the request
- Way to handle single requests from multiple handlers.
Design Patterns Book
Design Patterns are one of the most famous solutions in programming and millions of people follow them to fix their tasks, design projects, and so on. Most of the patterns are created based on basic OOP concepts (If your beginner then read our basic OOP, Encapsulation, Inheritance, Polymorphism, and Abstraction articles with great examples )
There are a lot of articles and design pattern books available with different examples to read. Other than our article if you are interested in this topic you could go through the below books as well.
Books | Description |
---|---|
Head First Design Patterns: A Brain-Friendly Guide | My favorite and it’s more readable. There are good examples and they look good to beginners as well. |
Design Patterns: Elements of Reusable Object-Oriented Software | There are 23 design patterns. showing how to select an appropriate pattern for your case. |
Head First Design Patterns: Building Extensible and Maintainable Object-Oriented Software | There are a lot of simple examples showing how to use design patterns in the correct way. Those are based on SOLIC principles. |
There are a lot of design pattern ebooks on Amazon. Those design patterns are written with great examples in a different programming language. I included a few of them according to their best ranking.