Bridge Design Pattern is one of the main Design Patterns focusing on decoupling abstraction from implementation. The concept helps to grow implementation and abstraction separately without coupling each other. Also, Bridge Design Pattern hides the implementation from clients.
Bridge Design Pattern comes under structural design patterns. It increases the loose couple between abstraction and implementation. Just use the bridge to do the connection between them. Let’s take an example now.
Bridge Design Pattern Real World Example
Consider Vehicle Paint Shop which provides services such as Painting and Polishing. Different types of vehicles come to the shop, but shops do not depend on the vehicle types just doing the paintings and polishing. In this case, has to decouple the vehicles and shop services. Let’s move on to the class diagram of the paint shop as a real-world problem.
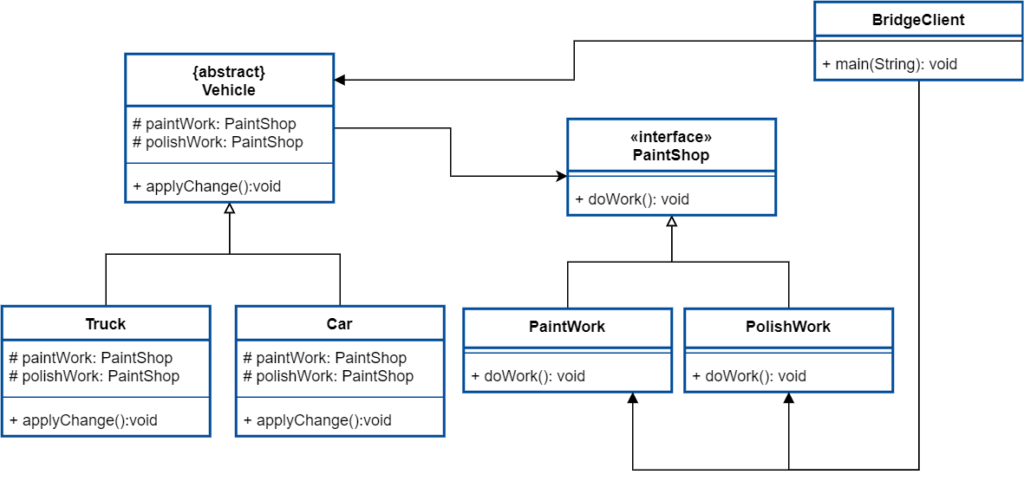
According to the diagram, the paint shop could have many services(Paintwork, Polish work), and vehicles come to the shop to get some services. According to the example services are independent and the abstract vehicle creates the connection between Vehicle and Paint Shop.
Bridge Design Pattern in Java
Bridge Design patterns can divide into the abstraction side and the implementation side. But According to the diagram, there are four main participants in the design pattern. Let’s see what are the participants in this pattern
- Abstraction: Define the functionality (PaintShop)
- Bridge: Connection between abstraction side and implementation side (Vehicle)
- Implementation: Implement the functionality defined by the abstraction. (PaintWork, PolishWork)
- Concrete Implementations: Use the Bridge and Complete the work using abstract implementation (Truck, Car)
// Define the work of Paint Shop interface PaintShop{ void doWork(); } //Implements the services that Paint shop Provide class PaintWork implements PaintShop{ @Override public void doWork(){ System.out.println("Paint Shop doing paint works"); } } class PolishWork implements PaintShop{ @Override public void doWork(){ System.out.println("Paint Shop doing polish works"); } } // Bridge the PaintShop and Vehicles abstract class Vehicle{ protected PaintShop paintWork; protected PaintShop polishWork; public Vehicle(PaintShop paintWork,PaintShop polishWork) { this.paintWork=paintWork; this.polishWork=polishWork; } public abstract void applyChange(); } // Get the required services from PaintShop class Truck extends Vehicle{ public Truck(PaintShop paintWork,PaintShop polishWork) { super(paintWork,polishWork); } @Override public void applyChange(){ System.out.println("Truck work going to start"); paintWork.doWork(); polishWork.doWork(); } } class Car extends Vehicle{ public Car(PaintShop paintWork,PaintShop polishWork) { super(paintWork,polishWork); } @Override public void applyChange(){ System.out.println("Car work going to start"); paintWork.doWork(); polishWork.doWork(); } } public class BridgeClient { public static void main(String[] args) { Vehicle vehicle1= new Truck(new PaintWork(), new PolishWork()); vehicle1.applyChange(); Vehicle vehicle2= new Car(new PaintWork(), new PolishWork()); vehicle2.applyChange(); } }
In this example, There are Trucks and Cars inherited from the Vehicle class. But there can be many vehicle types. On another side, there is an interface as PaintShop and there are PaintWork and PolishWork inherited from PaintShop. New services are able to add to the paint shop without affecting the system.
Output
Truck work going to start Paint Shop doing paint works Paint Shop doing polish works Car work going to start Paint Shop doing paint works Paint Shop doing polish works
Advantages of Bridge Design Pattern
- Bridge Design Pattern use to decouple an abstraction from implementation.
- Hide the implementation from clients.
- Possible to individually improve the interface side and implementation.
- Possible to implement as runtime binding objects.
Design Patterns Book
Design Patterns are one of the most famous solutions in programing and millions of people follow them to fix their tasks, design projects, and so on. Most of the patterns create based on basic OOP concepts (If your beginner then read our basic OOP, Encapsulation, Inheritance, Polymorphism, and Abstraction articles with great examples )
There are a lot of articles and design pattern books available with different examples to read. Other than our article if you are interested in this topic you could go through the below books as well.
Books | Description | |
---|---|---|
Head First Design Patterns: A Brain-Friendly Guide | My favorite and it’s more readable. There are good examples and they look good to beginners as well. | |
Design Patterns: Elements of Reusable Object-Oriented Software | There are 23 design patterns. showing how to select an appropriate pattern for your case. | |
Head First Design Patterns: Building Extensible and Maintainable Object-Oriented Software | There are a lot of simple examples showing how to use design patterns in the correct way. Those are based on SOLIC principles. |
There are a lot of design pattern ebooks on Amazon. Those design patterns are written with great examples in a different programming language. I included a few of them according to their best ranking.